ECMAScript 5 and JS frameworks
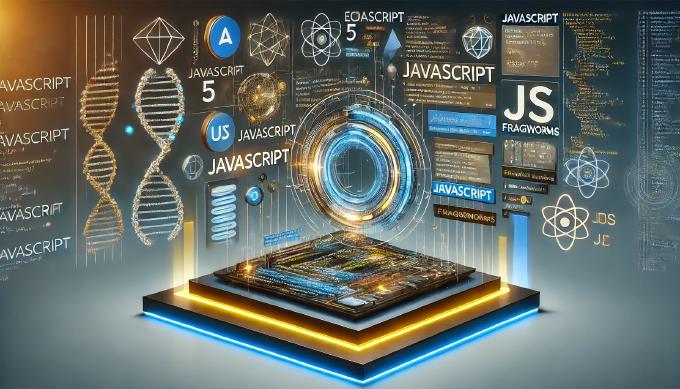
Standards are being always approved with a slow pace. Though, the features declared in drafts usually find their implementation long before the standard release. Let’s take for an instance Websockets, which were already implemented in Chrome/Safari and Firefox 4b when standard unexpectedly changed. Apparently YUI3 and jQuery have implemented for long time the features, which are only coming up with ECMAScript 5 (the implementations is called JavaScript 1.8.5) spread.
YUI 3 from the very beginning introduced controllable attributes. It is used like that:
...
var attributeConfig = {
attrA : {
// Configuration for attribute "attrA"
value: 5,
setter: function(val) {
return Math.min(val, 10);
},
validator: function(val) {
return Y.Lang.isNumber(val);
}
},
attrB : {
// Configuration for attribute "attrB"
}
};
this.addAttrs(attributeConfig, userValues);
...
Or
this.addAttr("attrA", {
// Configuration for attribute "attrA"
});
Now look at ECMA 5 code:
var objA = Object.create(ClassA, {
attrA : {
get : function () { /* . . . */ },
set : function (val) { /* . . . */ },
enumerable: true
},
attrB : {
// Configuration for attribute "attrB"
}
});
or
Object.defineProperty(ClassA, " attrA ", {
// Configuration for attribute "attrA"
});
New methods of ECMAScript 5 such as Array.isArray, Array.map, Array.filter, Array.trim are implemented to jQuery almost identically.
For the method Function.bind, jQuery has analog $.proxy. Just compare:
var funcA = function() { .. };
jQuery.proxy(funcA, this);
and
var funcA = function() { .. };
funcA.bind(this);
So, what about frameworks? Shouldn’t we use these features natively now instead? It seems not yet. They are implemented only in Firefox 4, IE 9, Safari 5 and Chrome 5+. I doubt that most of your users have installed those versions already. But some day… Some day JS will meet harmony.